PyQT5 输入对话框
PyQt5 支持几个输入对话框,使用它们导入 QInputDialog
。
from PyQt5.QtWidgets import QApplication, QWidget, QInputDialog, QLineEdit
PyQt5 输入对话框概述 :
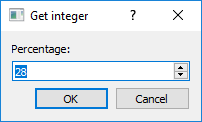
获取整数
使用 QInputDialog.getInt()
获取整数 :
def getInteger(self):
i, okPressed = QInputDialog.getInt(self, "Get integer","Percentage:", 28, 0, 100, 1)
if okPressed:
print(i)
按顺序排列的参数:self,窗口标题,标签(输入框之前),默认值,最小值,最大值和步长。
获取双精度小数
使用 QInputDialog.getDouble()
获取双精度小数:
def getDouble(self):
d, okPressed = QInputDialog.getDouble(self, "Get double","Value:", 10.05, 0, 100, 10)
if okPressed:
print(d)
最后一个参数 10
是逗号后面的小数位数。
获取元素/选择
从下拉框中获取元素:
def getChoice(self):
items = ("Red","Blue","Green")
item, okPressed = QInputDialog.getItem(self, "Get item","Color:", items, 0, False)
if okPressed and item:
print(item)
获取字符串
字符串使用 QInputDialog.getText()
来获取。
def getText(self):
text, okPressed = QInputDialog.getText(self, "Get text","Your name:", QLineEdit.Normal, "")
if okPressed and text != '':
print(text)
PyQt5 输入对话框举例
示例完整示例如下:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QInputDialog, QLineEdit
from PyQt5.QtGui import QIcon
class App(QWidget):
def __init__(self):
super().__init__()
self.title = 'PyQt5 input dialogs - tastones.com'
self.left = 10
self.top = 10
self.width = 640
self.height = 480
self.initUI()
def initUI(self):
self.setWindowTitle(self.title)
self.setGeometry(self.left, self.top, self.width, self.height)
self.getInteger()
self.getText()
self.getDouble()
self.getChoice()
self.show()
def getInteger(self):
i, okPressed = QInputDialog.getInt(self, "Get integer","Percentage:", 28, 0, 100, 1)
if okPressed:
print(i)
def getDouble(self):
d, okPressed = QInputDialog.getDouble(self, "Get double","Value:", 10.50, 0, 100, 10)
if okPressed:
print( d)
def getChoice(self):
items = ("Red","Blue","Green")
item, okPressed = QInputDialog.getItem(self, "Get item","Color:", items, 0, False)
if ok and item:
print(item)
def getText(self):
text, okPressed = QInputDialog.getText(self, "Get text","Your name:", QLineEdit.Normal, "")
if okPressed and text != '':
print(text)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = App()
sys.exit(app.exec_())