PyQt5 拖放
与任何现代 GUI 工具包一样,PyQt5 支持拖放。必须使用 setDragEnabled(True)
方法调用设置 widget 参数。然后应将自定义窗口小控件设置为接受带有 setAcceptDrops(True)
的拖放。
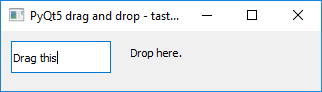
PyQt5 拖放示例
将文本从输入字段拖到标签,标签将更新其文本。
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLineEdit, QLabel
from PyQt5.QtGui import QIcon
from PyQt5.QtCore import pyqtSlot
class App(QWidget):
def __init__(self):
super().__init__()
self.title = 'PyQt5 drag and drop - tastones.com'
self.left = 10
self.top = 10
self.width = 320
self.height = 60
self.initUI()
def initUI(self):
self.setWindowTitle(self.title)
self.setGeometry(self.left, self.top, self.width, self.height)
editBox = QLineEdit('Drag this', self)
editBox.setDragEnabled(True)
editBox.move(10, 10)
editBox.resize(100,32)
button = CustomLabel('Drop here.', self)
button.move(130,15)
self.show()
@pyqtSlot()
def on_click(self):
print('PyQt5 button click')
class CustomLabel(QLabel):
def __init__(self, title, parent):
super().__init__(title, parent)
self.setAcceptDrops(True)
def dragEnterEvent(self, e):
if e.mimeData().hasFormat('text/plain'):
e.accept()
else:
e.ignore()
def dropEvent(self, e):
self.setText(e.mimeData().text())
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = App()
sys.exit(app.exec_())
使用调用 QLineEdit()
创建文本框。创建一个接受拖放的自定义类 CustomLabel
。这两个事件都被定义为方法,并在事件发生时执行其逻辑。