PyQt5 繪畫和畫素
你可以使用 QPainter 小控制元件在 PyQt5 視窗中繪製。與其他小控制元件不同,此小控制元件支援在小控制元件內新增畫素(點)。在本文中,我們將解釋如何在 Python 中使用 QPainter 小控制元件。
要在 Qt5 中使用小控制元件,我們匯入 PyQt5.QtGui
。這還包含其他類,如 QPen
和 QColor
。
QPainter
小控制元件示例
我們使用以下方法設定視窗背景:
# Set window background color
self.setAutoFillBackground(True)
p = self.palette()
p.setColor(self.backgroundRole(), Qt.white)
self.setPalette(p)
使用 drawPoint(x,y)
方法新增畫素。
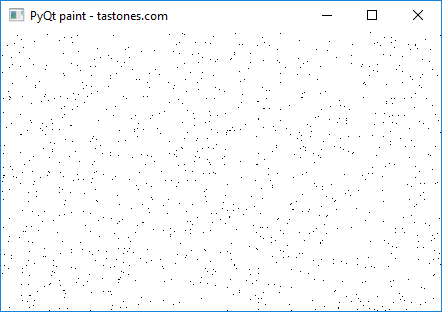
PyQt5 QPainter 示例
下面的示例描繪了 QPainter 小控制元件中的畫素:
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QMainWindow, QLabel
from PyQt5.QtGui import QPainter, QColor, QPen
from PyQt5.QtGui import QIcon
from PyQt5.QtCore import Qt
import random
class App(QMainWindow):
def __init__(self):
super().__init__()
self.title = 'PyQt paint - tastones.com'
self.left = 10
self.top = 10
self.width = 440
self.height = 280
self.initUI()
def initUI(self):
self.setWindowTitle(self.title)
self.setGeometry(self.left, self.top, self.width, self.height)
# Set window background color
self.setAutoFillBackground(True)
p = self.palette()
p.setColor(self.backgroundRole(), Qt.white)
self.setPalette(p)
# Add paint widget and paint
self.m = PaintWidget(self)
self.m.move(0,0)
self.m.resize(self.width,self.height)
self.show()
class PaintWidget(QWidget):
def paintEvent(self, event):
qp = QPainter(self)
qp.setPen(Qt.black)
size = self.size()
for i in range(1024):
x = random.randint(1, size.width()-1)
y = random.randint(1, size.height()-1)
qp.drawPoint(x, y)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = App()
sys.exit(app.exec_())