PyQt5 拖放
與任何現代 GUI 工具包一樣,PyQt5 支援拖放。必須使用 setDragEnabled(True)
方法呼叫設定 widget 引數。然後應將自定義視窗小控制元件設定為接受帶有 setAcceptDrops(True)
的拖放。
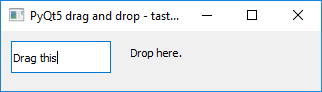
PyQt5 拖放示例
將文字從輸入欄位拖到標籤,標籤將更新其文字。
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QLineEdit, QLabel
from PyQt5.QtGui import QIcon
from PyQt5.QtCore import pyqtSlot
class App(QWidget):
def __init__(self):
super().__init__()
self.title = 'PyQt5 drag and drop - tastones.com'
self.left = 10
self.top = 10
self.width = 320
self.height = 60
self.initUI()
def initUI(self):
self.setWindowTitle(self.title)
self.setGeometry(self.left, self.top, self.width, self.height)
editBox = QLineEdit('Drag this', self)
editBox.setDragEnabled(True)
editBox.move(10, 10)
editBox.resize(100,32)
button = CustomLabel('Drop here.', self)
button.move(130,15)
self.show()
@pyqtSlot()
def on_click(self):
print('PyQt5 button click')
class CustomLabel(QLabel):
def __init__(self, title, parent):
super().__init__(title, parent)
self.setAcceptDrops(True)
def dragEnterEvent(self, e):
if e.mimeData().hasFormat('text/plain'):
e.accept()
else:
e.ignore()
def dropEvent(self, e):
self.setText(e.mimeData().text())
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = App()
sys.exit(app.exec_())
使用呼叫 QLineEdit()
建立文字框。建立一個接受拖放的自定義類 CustomLabel
。這兩個事件都被定義為方法,並在事件發生時執行其邏輯。